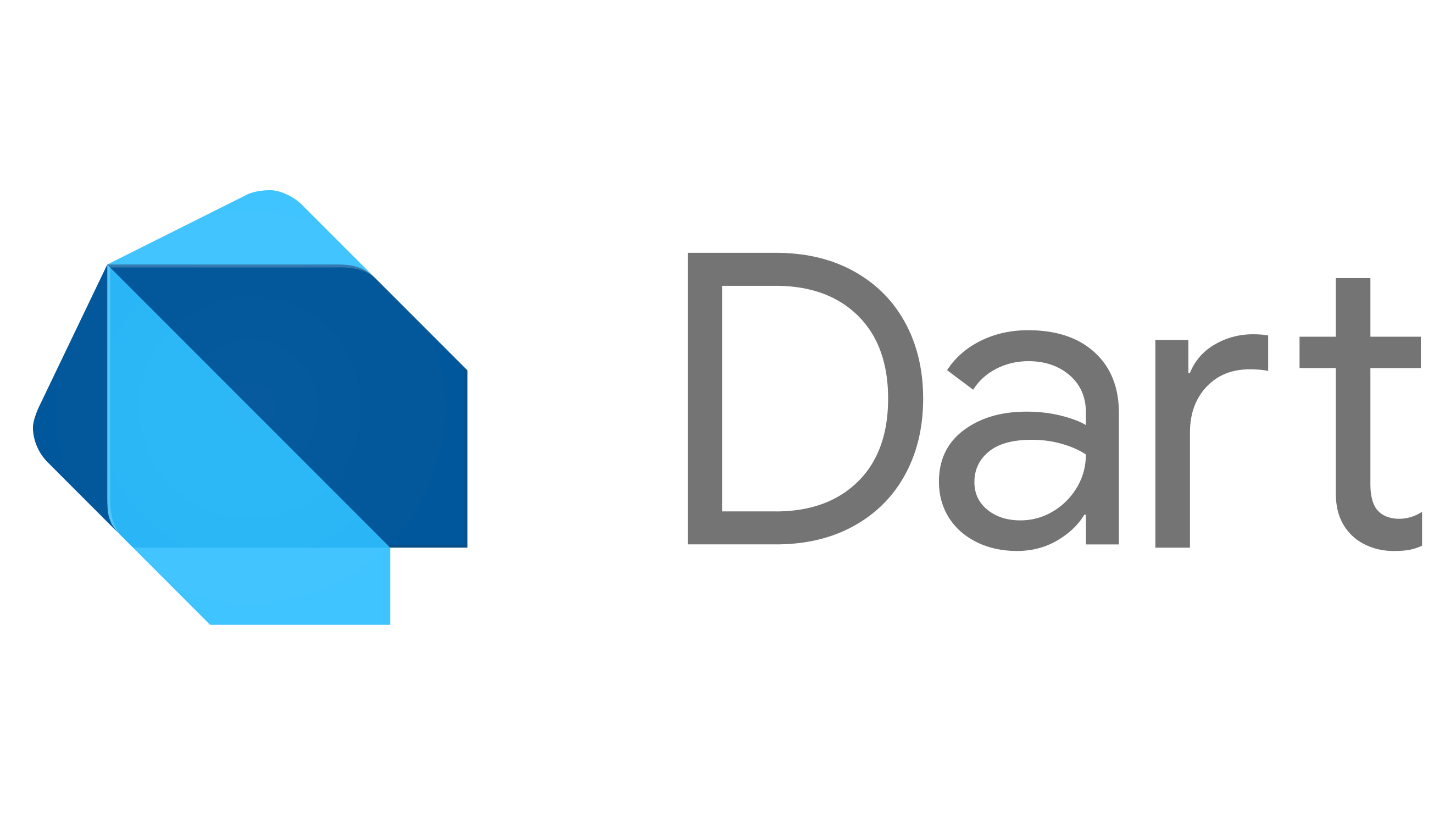
map
map은 forEach와 유사하게 동작한다. map과 forEach는 List의 element를 e변수에 차례대로 받아오고 element 값을 자리의 파라미터 값을 중괄호 안쪽의 기능수행 영역에서 사용할 수 있다. 다만 map 함수는 return 값을 반환할 수 있고 반환된 모든 값을 차례대로 변수에 저장할 수 있다는 차이가 있다.
void main() {
List<String> colors = ['red', 'orange', 'yellow', 'green', 'blue'];
// forEach loop
colors.forEach((e) {
print(e);
});
// map loop
final result = colors.map((e){
return '${e} is color';
});
}
하지만 map의 반환 결과는 List 타입이 아니므로 toList 메소드를 이용하여 타입 캐스팅을 해주어야 한다.
void main() {
List<String> colors = ['red', 'orange', 'yellow', 'green', 'blue'];
final result = colors.map((e){
return '${e} is color';
});
print(result.runtimeType); // MappedListIterable<String, String>
print(result); // (red is color, orange is color, yellow is color, green is color, blue is color)
List newList = result.toList();
print(newList); // [red is color, orange is color, yellow is color, green is color, blue is color]
}
reduce
reduce는 iterable의 요소를 하나씩 꺼내서 연산을 하는데 그 결과를 누적하여 반환해 주는 메소드이다. 쉽게 말해 List의 첫 번째 요소부터 연산된 결과를 누적하여 반복연산하는 기능을 수행한다. reduce는 실행하는 리스트의 멤버들의 타입과 반환하는 타입이 같아야한다.
void main() {
final numbers = <int>[2, 3, 4];
final result1 = numbers.reduce((prev, next) => prev + next);
final result2 = numbers.reduce((prev, next) => prev * next);
print(result1); // 9
print(result2); // 24
}
fold
fold는 시작값, value, element 이렇게 세 개의 매개변수를 가진다. reduce의 기능과 유사하지만 List의 첫 요소부터 누적 연산이 아니라 초기값을 지정할 수 있는 함수이다. reduce와 다르게 fold는 반환되는 타입을 지정할 수 있어 유연하게 사용할 수 있다.
void main() {
final numbers = <int>[1, 2, 3];
const initialValue = 100.5;
final result = numbers.fold<double>(initialValue, (prev, next) => prev + next);
print(result); // 106.5
}
관련 포스트
2024.04.08 - [Languages/Flutter(Dart)] - [Dart] 외부반복(for)과 내부반복(forEach)
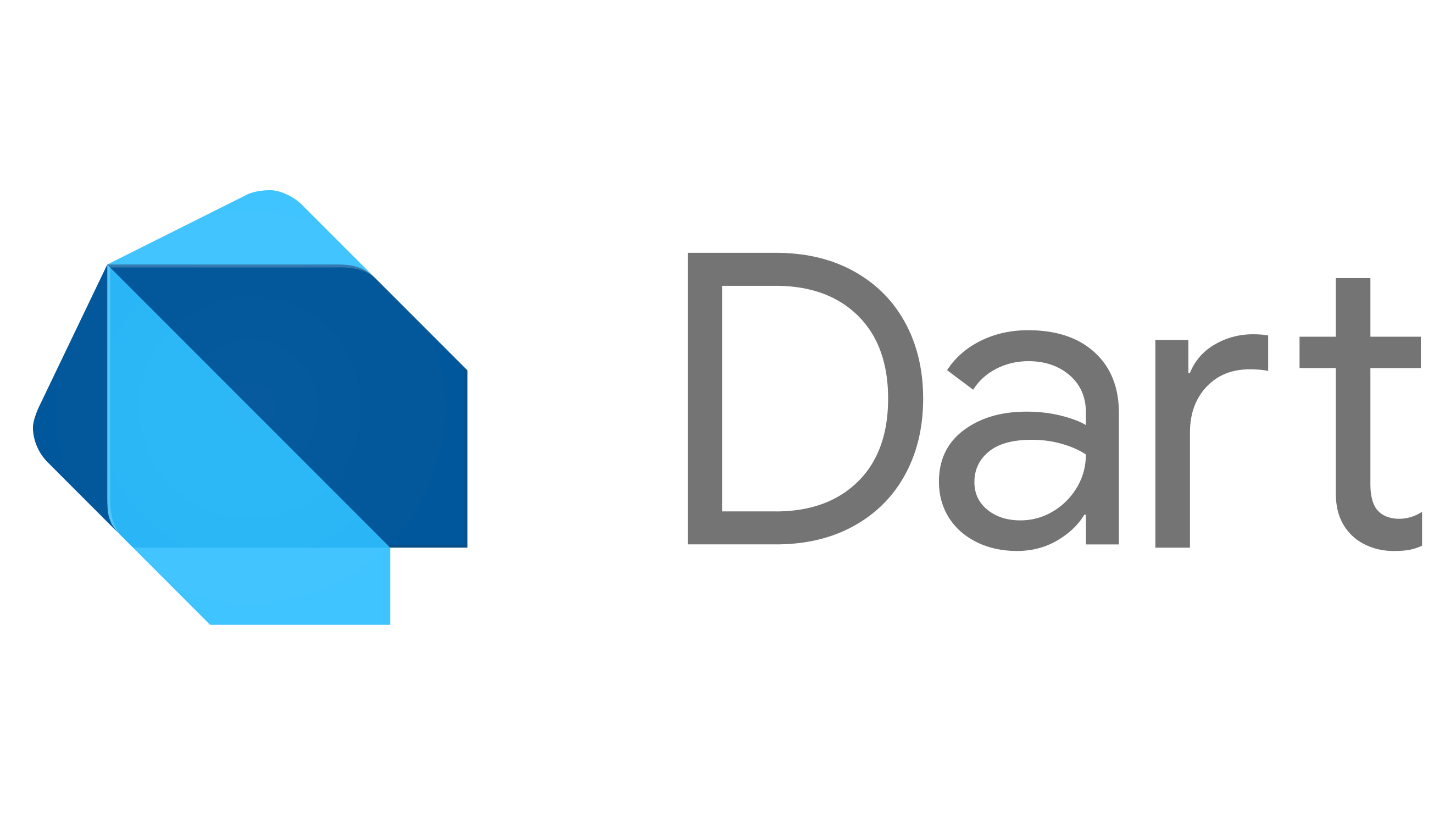
map
map은 forEach와 유사하게 동작한다. map과 forEach는 List의 element를 e변수에 차례대로 받아오고 element 값을 자리의 파라미터 값을 중괄호 안쪽의 기능수행 영역에서 사용할 수 있다. 다만 map 함수는 return 값을 반환할 수 있고 반환된 모든 값을 차례대로 변수에 저장할 수 있다는 차이가 있다.
void main() {
List<String> colors = ['red', 'orange', 'yellow', 'green', 'blue'];
// forEach loop
colors.forEach((e) {
print(e);
});
// map loop
final result = colors.map((e){
return '${e} is color';
});
}
하지만 map의 반환 결과는 List 타입이 아니므로 toList 메소드를 이용하여 타입 캐스팅을 해주어야 한다.
void main() {
List<String> colors = ['red', 'orange', 'yellow', 'green', 'blue'];
final result = colors.map((e){
return '${e} is color';
});
print(result.runtimeType); // MappedListIterable<String, String>
print(result); // (red is color, orange is color, yellow is color, green is color, blue is color)
List newList = result.toList();
print(newList); // [red is color, orange is color, yellow is color, green is color, blue is color]
}
reduce
reduce는 iterable의 요소를 하나씩 꺼내서 연산을 하는데 그 결과를 누적하여 반환해 주는 메소드이다. 쉽게 말해 List의 첫 번째 요소부터 연산된 결과를 누적하여 반복연산하는 기능을 수행한다. reduce는 실행하는 리스트의 멤버들의 타입과 반환하는 타입이 같아야한다.
void main() {
final numbers = <int>[2, 3, 4];
final result1 = numbers.reduce((prev, next) => prev + next);
final result2 = numbers.reduce((prev, next) => prev * next);
print(result1); // 9
print(result2); // 24
}
fold
fold는 시작값, value, element 이렇게 세 개의 매개변수를 가진다. reduce의 기능과 유사하지만 List의 첫 요소부터 누적 연산이 아니라 초기값을 지정할 수 있는 함수이다. reduce와 다르게 fold는 반환되는 타입을 지정할 수 있어 유연하게 사용할 수 있다.
void main() {
final numbers = <int>[1, 2, 3];
const initialValue = 100.5;
final result = numbers.fold<double>(initialValue, (prev, next) => prev + next);
print(result); // 106.5
}
관련 포스트
2024.04.08 - [Languages/Flutter(Dart)] - [Dart] 외부반복(for)과 내부반복(forEach)